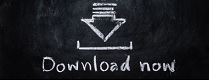
Here is a doubly linked list Structure syntax: struct node. Nevertheless, because of the extra pointer in each node, they require more memory than singly-linked lists.
LINKED LIST IN PYTHON CODE
> from collections import deque > deque() deque ( ) The code above will create an empty linked list. In this article, using the strength of Object-Oriented Programming (OOP) ideas, we examine the idea of a Doubly Linked List in Python. You can use the following piece of code to do that with deque: >. You can imagine a linked list as a chain where each link is connected to the next one to form a sequence with a start and an end. They have various advantages over singly-linked lists, including the ability to traverse the list in both ways, which makes them efficient for some algorithms. However, in this article you’ll only touch on a few of them, mostly for adding or removing elements. Each node in the list contains a data element as well as two pointers to the previous and next nodes in the sequence.ĭoubly linked lists are handy data structures for implementing lists, queues, and stacks, among other data structures. node linkedlist.head while node: print node.value node node. The next step is to join multiple single nodes containing data using the next pointers, and have a single head pointer pointing to a complete instance of a Linked List. And the final node is connected to a NULL reference in the next pointer because there is no node after it. You can use a while loop, setting a variable to the head at first and the next node on each iteration. In a doubly linked list, the first node is connected to a NULL reference in the previous pointer because there is no node before it. This enables the traversal of the list in both forward and backward directions.
LINKED LIST IN PYTHON MANUAL
There are manual ways to make your function behave in a tail-call-optimized fashion, even if Python won't do it for you, but they are complex and it is by far simpler to just use a loop. This means that if your linked-list is very long, you will get a stack overflow. When the list is empty, the head pointer points. Both use recursion, and Python does not do tail-call-optimization. The head point means the first node, and the last element in the list points to null. Each node includes both data and a reference to the next node in the list.
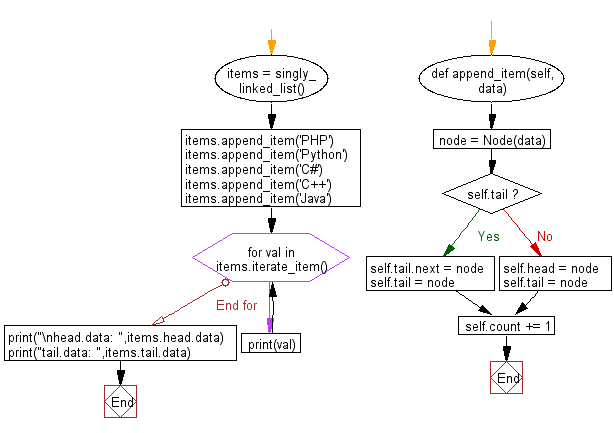
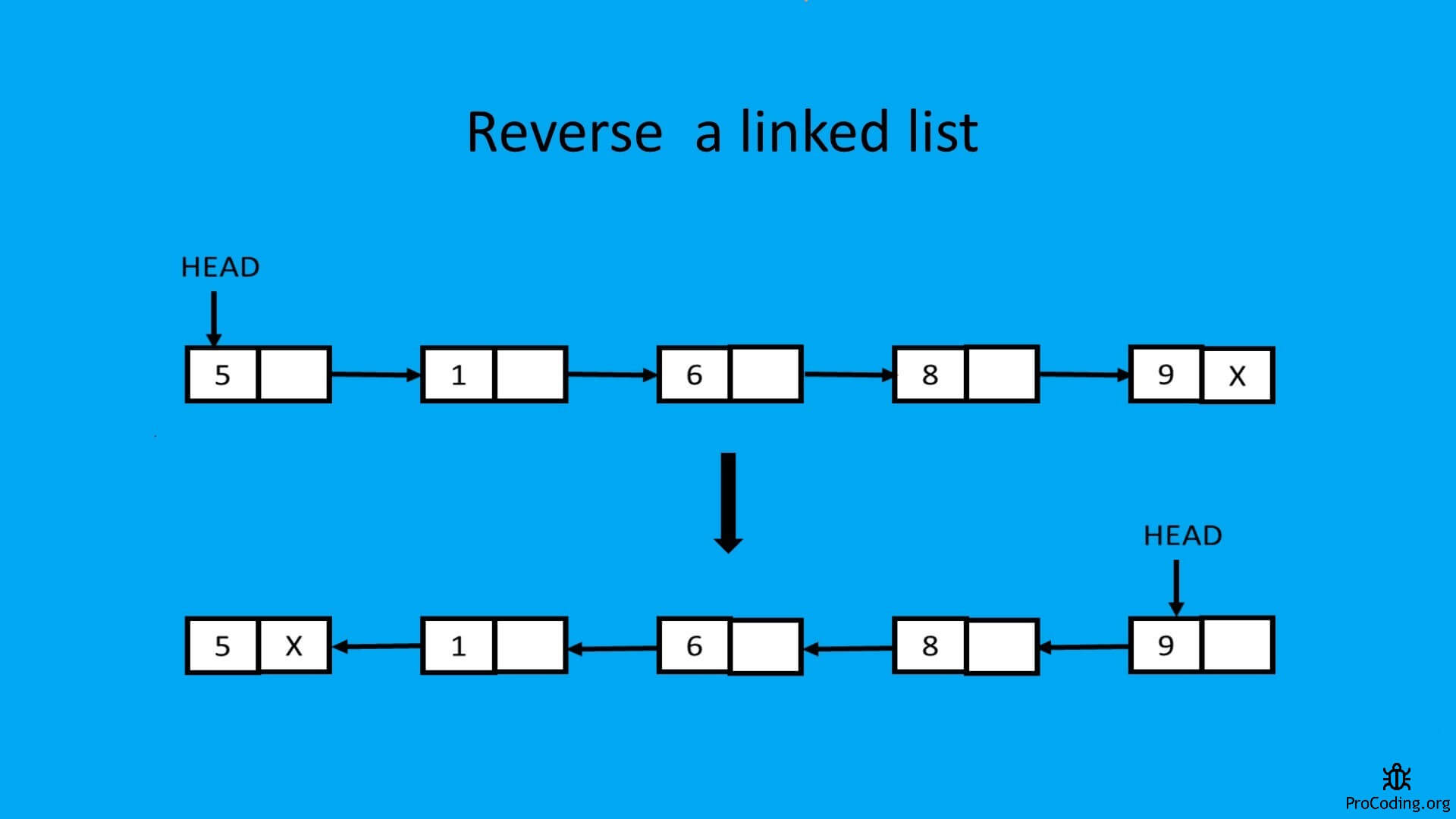
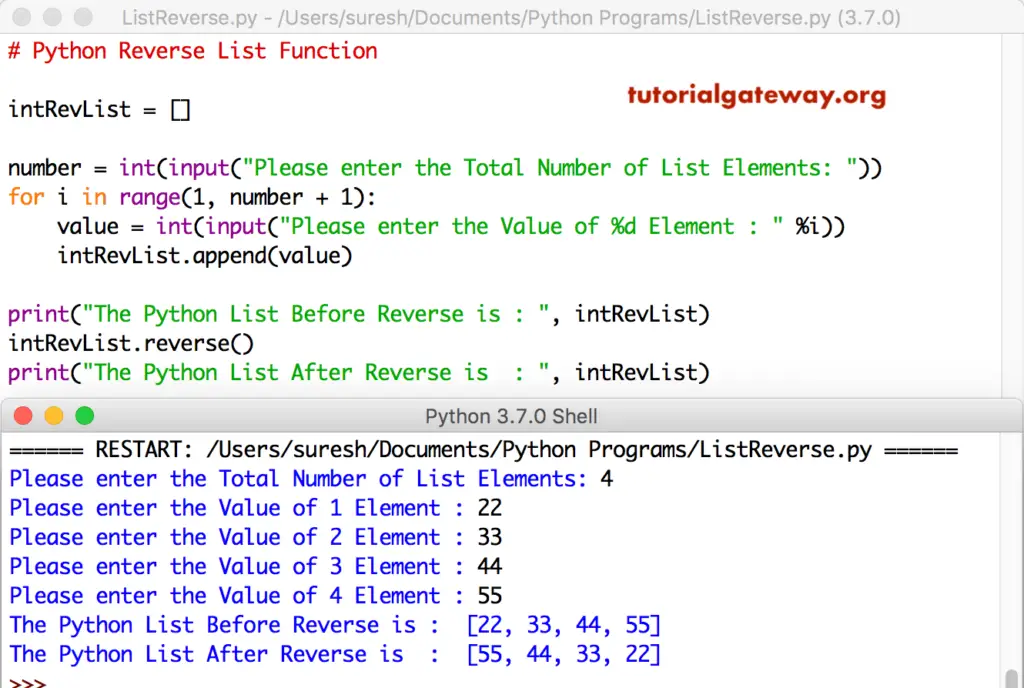
A doubly linked list is a kind of linked list in which each node has two pointers or references: one to the previous node in the sequence and one to the next node in the series. What is Linked List in Python The Linked List is a linear data structure made of multiple objects called nodes with a common data type.
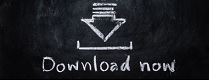